REST API Basic Guide
This document will describe the basic usage of MyQ REST API. Its purpose is to prepare 3rd party software and services for integration with MyQ X.
You will learn how to create a REST API application in MyQ Web UI and use generated credentials to get an Authentication token from the server, and perform basic operations.
You should have already installed MyQ Print Server, and if not, see the Installation guide.
REST API Purpose and Usage
Example of possible integrations:
Print portals and intranets.
Recharging MyQ credit, quota.
Managing user profiles.
PIN operations.
Managing jobs.
Remote storage, including proprietary services or local storage not natively supported by MyQ X.
Storage to print from with Easy Print.
Storage for delivering scans from Easy Scan.
Remote run and download of MyQ reports.
…and more.
Documentation and YAML files
The full technical specification of the REST API is provided in YAML files with every MyQ X installation. These files contain the description of all available API endpoints, as well as their requirements (scopes, token type), and usage.
Read how to obtain the files and read them here.
Setup in Web Interface
Register Your Client
First, you have to create a client and assign it permissions (scopes) to allow it to interact with MyQ X.
In the MyQ X Web Interface:
Open Settings tab.
Navigate to External Systems (or Connections).
Under REST API applications, click Add.
Specify the name and scopes required by your application.
Copy your Client ID and Secret; you will later need them to get an Authentication token from the X Server.
Click OK.
Before MyQ X 10.1, the Settings tab was called External Systems. From versions 10.1, this option is called Connections.
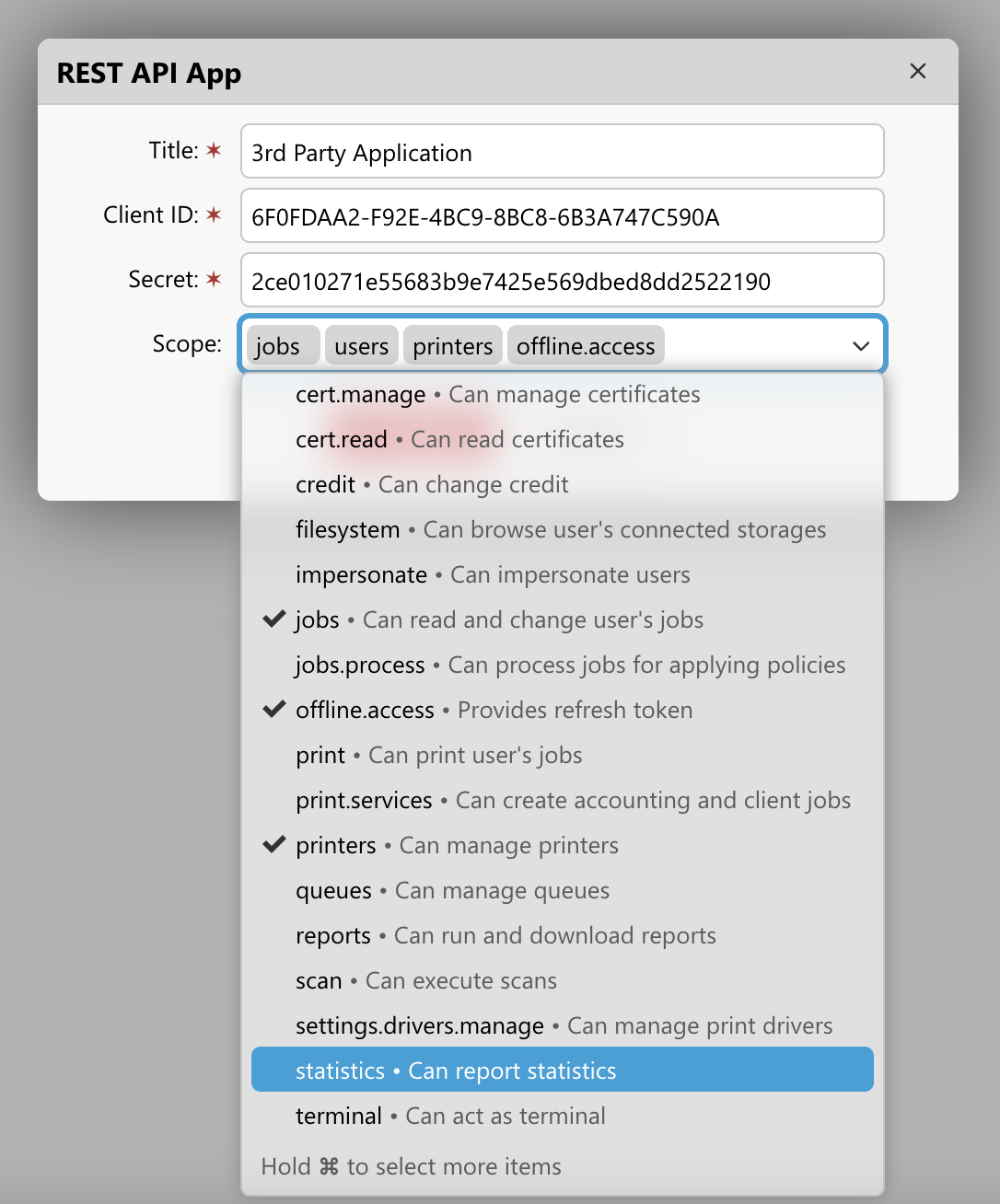
For testing purposes, you can assign all available scopes.
For production applications, select only those that your application requires to perform the desired operation as per the documentation.
Working with REST API
In this guide, we will use Postman to illustrate the features of the REST API.
You can download it from https://www.getpostman.com/apps.
Secure Connection
MyQ API requires a secure SSL connection and cannot run without it; a certificate must be installed on the client side for the communication to work.
For manual installation of the self-signed MyQ certificate:
If you are using a built-in CA from MyQ, export the CA certificate from the MyQ X Web Interface, in Settings > Network. If you are using your company’s CA or manual certificate management, you must obtain the CA certificate yourself from your organization.
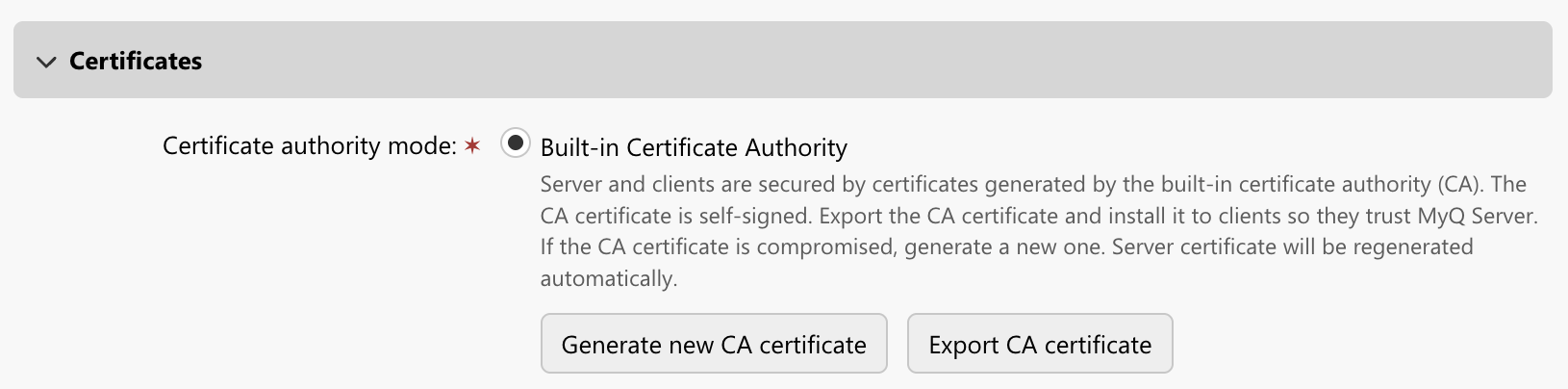
Another option is to copy the certificates (files server.cer
and myq-ca.cer
) from C:\ProgramData\MyQ\Cert
on your server.
Install the CA certificate; double-click the .cer file, select Install certificate > Local machine > Place all certificates in the following store (Browse) > Trusted Root Certification Authorities.
In Postman, you may need to first upload the CA certificate, so that the tool can verify the MyQ X’s server certificate during the initial steps of the connection.
For testing purposes, since we use a self-signed certificate, we can also temporarily configure Postman to skip this validation in File > Settings > General > SSL certificate verification > Off.

Client Authorization
To retrieve your Authentication token, you need to call the endpoint: POST /api/auth/token
with the request body specified below.
Create a new request. Go to the Authorization tab, and select:
Type to OAuth 2.0.
Add auth data to: Request Headers.
Header Prefix:
Bearer
.Grant Type: Client Credentials.
Paste the Access Token URL.
CODEhttps://serverHostname:webServerPort/api/auth/token
Replace the
serverHostname
andwebServerPort
with the values from your installation.Client ID, and Secret you acquired in MyQ X’s External Systems/Connections.
List all required Scopes, such as
users scan printers credit statistics offline.access
etc.Client Authentication: Send as Basic Auth header.
For more details about the grant types, refer to:
Print Server: Authentication Methods.
Central Server: Authentication Methods.
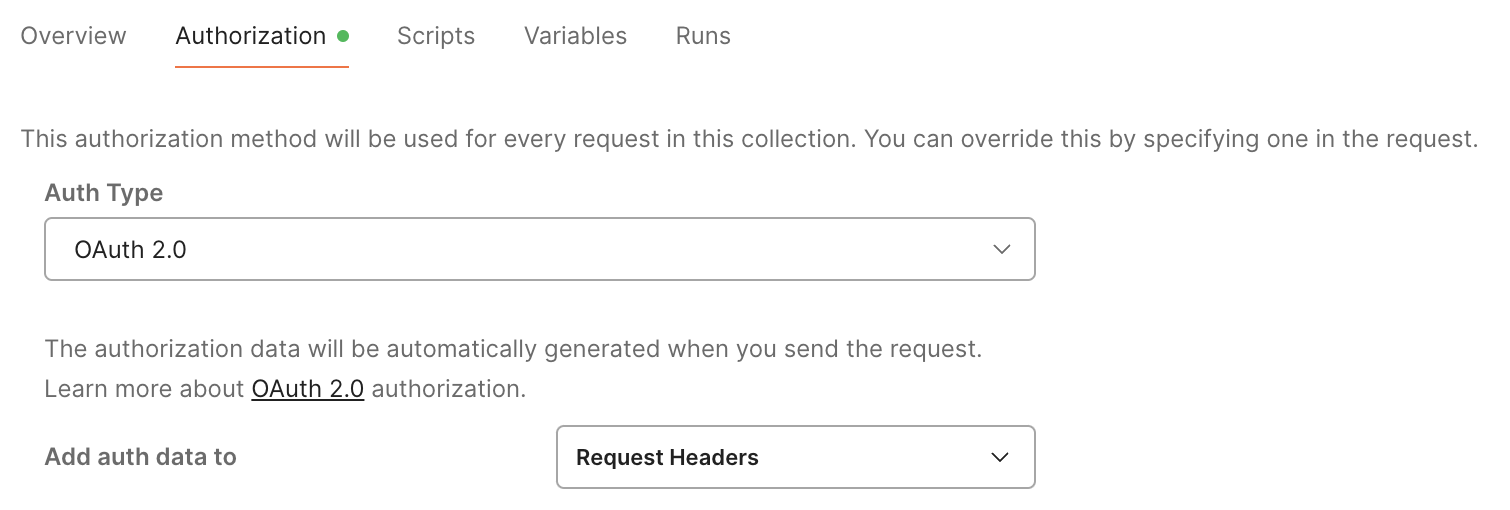

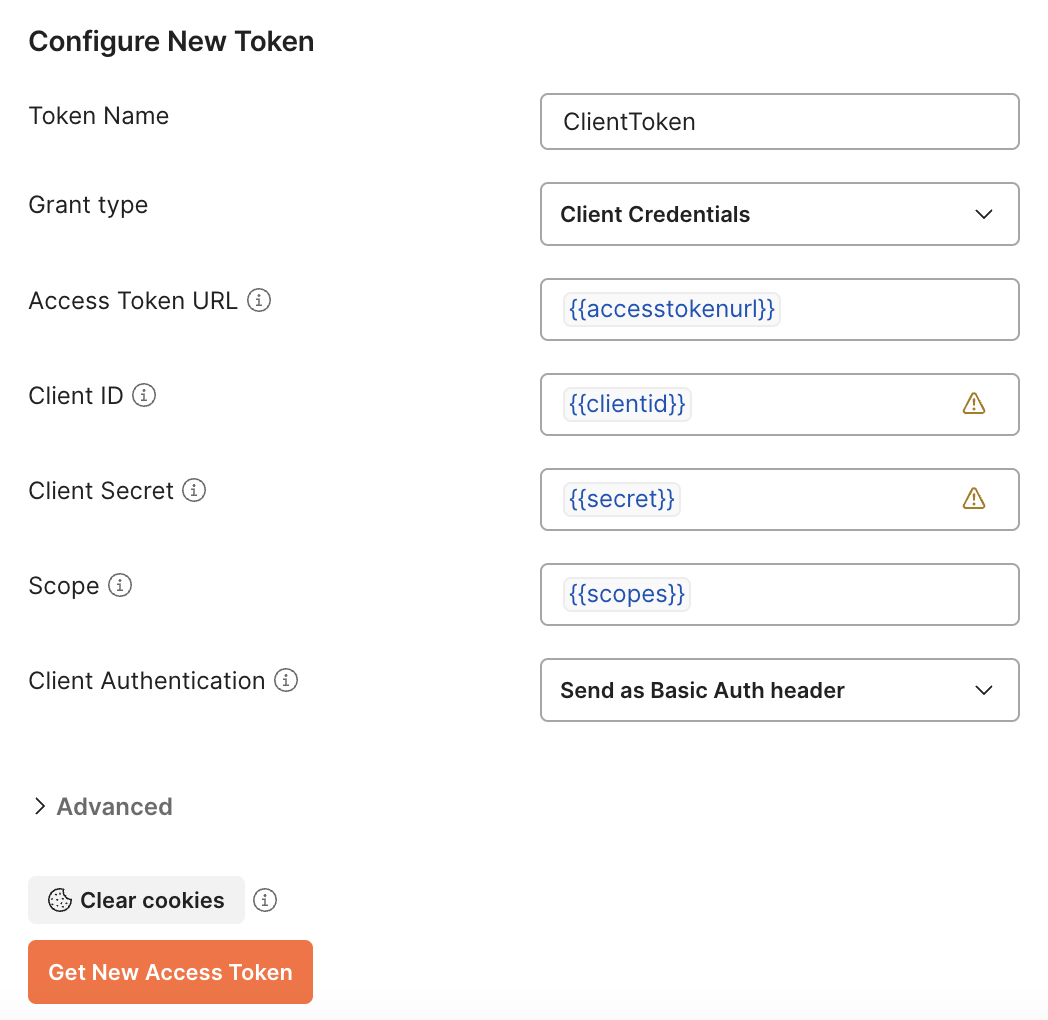
Click Get New Access Token.
If authentication happens, you'll receive an access token that must be provided at any other API endpoint.
Review the scopes and whether they align with the scopes you requested (Scopes in the Authorization request above), and if they do, click Use Token.
Example request:
POST https://print.acme.com:443/api/auth/token
{
"grant_type": "client_credentials",
"scope": "users scan printers credit statistics offline.access",
"client_id": "7B4CD3C2-F57E-4D52-A90A-23EED001CE81",
"client_secret": "89fbf537fe689fca26f67abae7a557106f4348d5"
}
Example response:
{
"access_token": "your_bearer_token",
"token_type": "Bearer",
"expires_in": 1800,
"scope": "users scan printers credit statistics offline.access",
"refresh_token": "your_refresh_token"
}
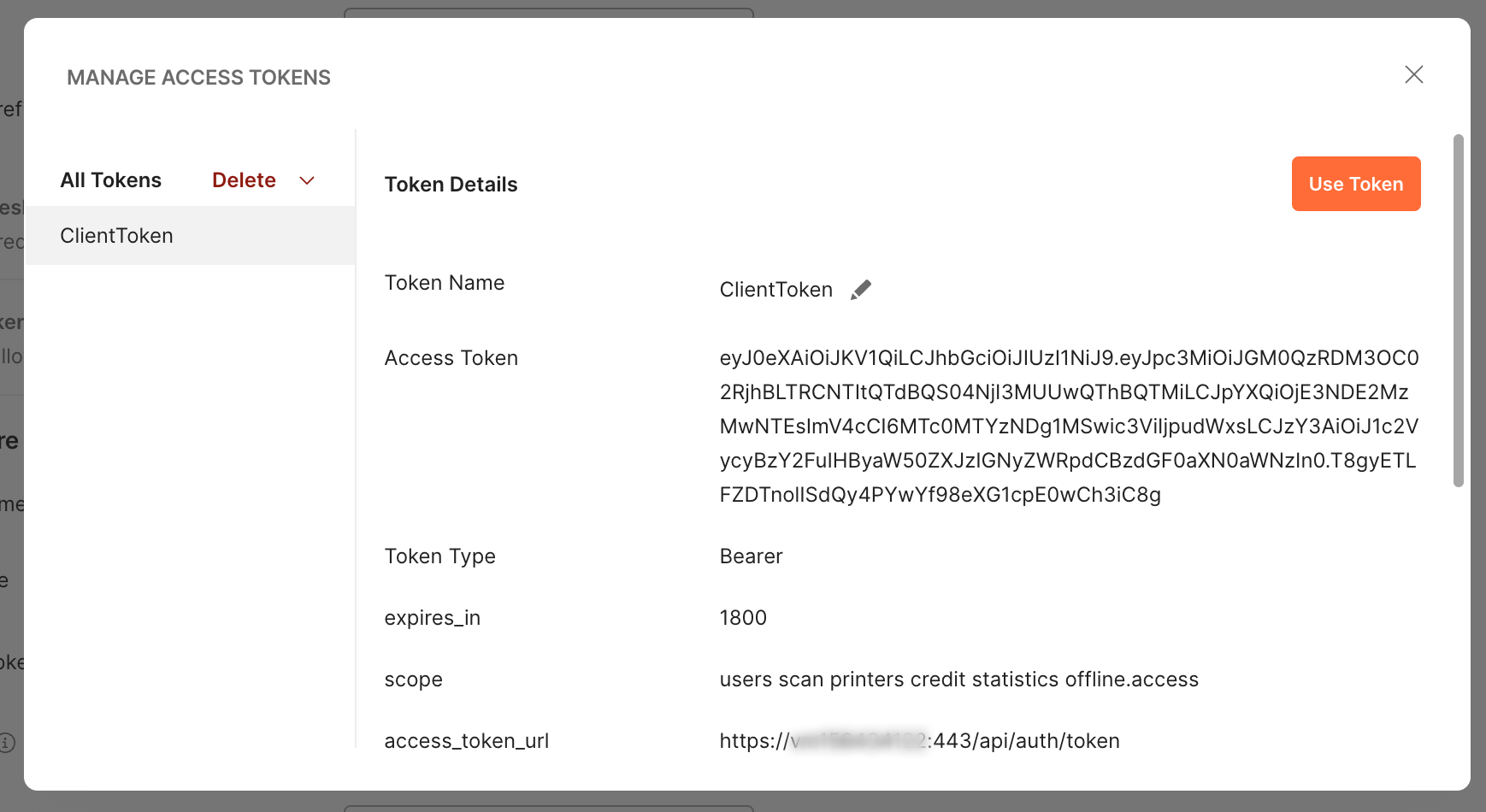
The client can be only granted scopes allowed in the REST API registration for this client ID in Settings > REST API App; otherwise, the server will return Invalid scopes
.
When the offline.access
scope is used, the client will also receive a refresh token that can be used to issue a new access token using the refresh_token
grant type.
Common Operations
Now we are ready to send our requests to the server using the client token from above.
Base URL
When we refer to the
{{baseUrl}
, we mean:https://serverHostname:webServerPort/api
Replace theserverHostname
andwebServerPort
with your values.
REST API version
The REST API version (e.g.,
v3
) that follows may differ between the Print Server and Central Server, read more at the end of this article.
Read Server Basic Configuration
GET {{baseUrl}}/v3/server/config
Required scope: none
At the beginning, it may be useful to obtain general information about the server such as the language, currency, printing methods available, supported job formats, accounting mode, and more.
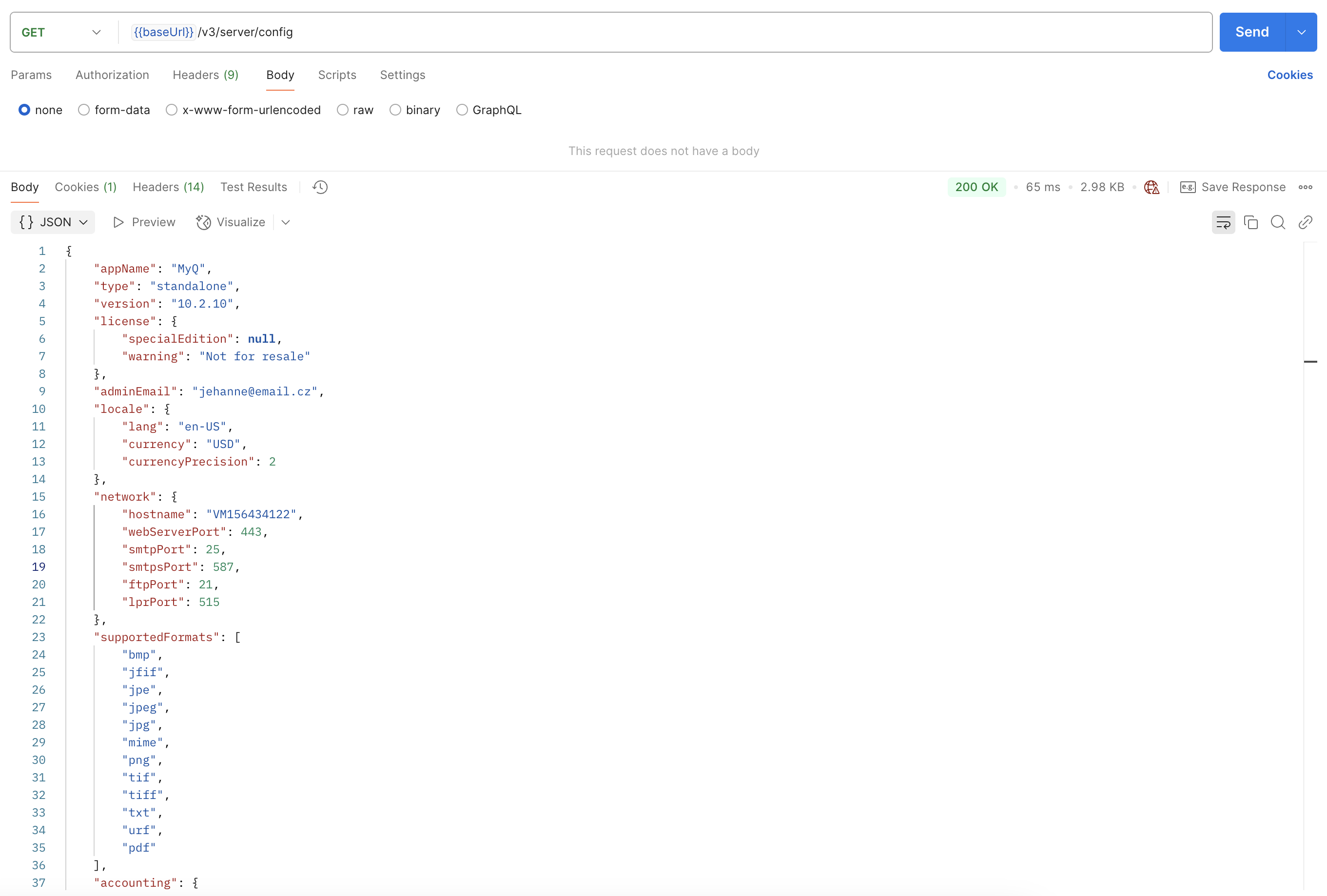
Obtain the User’s Information and ID
GET {{baseUrl}}/v3/users/find?username={{username}}&include=aliases,accounts
Required scope:
users
Let’s obtain a user’s ID by searching them by username.
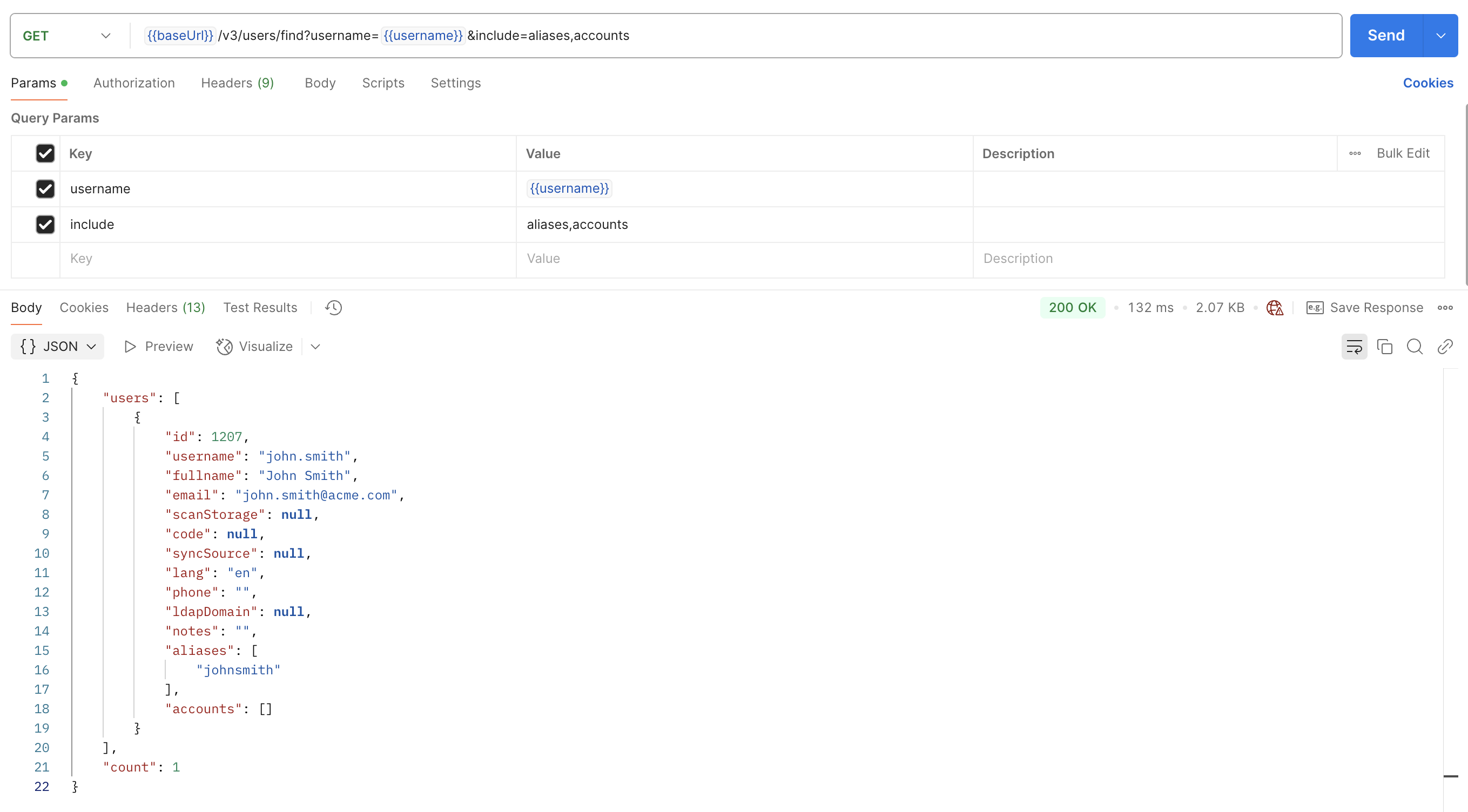
Example request:
POST {{baseUrl}}/v3/users/find?username=john.smith&include=aliases,accounts
As illustrated above, some endpoints also support the include
operation that requests additional information otherwise not included in the default response.
IDs are most commonly used to identify objects you want to perform operations on (user ID, printer ID, etc.).
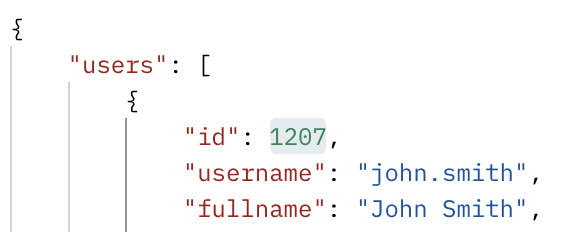
Request User Credit Balance
GET {{baseUrl}}/v3/users/{{userid}}/accounts
Required scope:
credit
And finally, we can request the user’s credit. In this request, you will utilize the user’s ID received above.
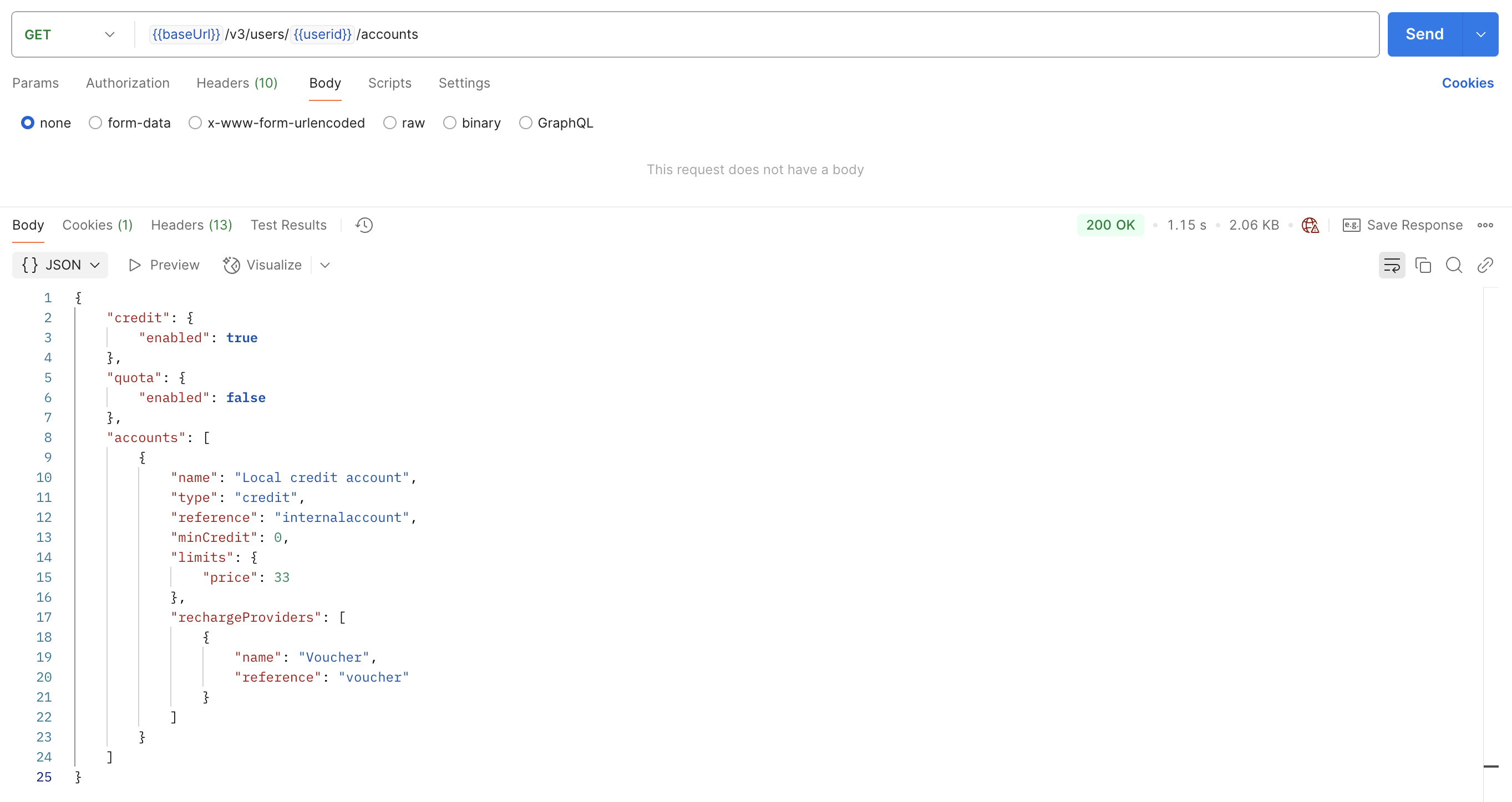
Example request:
POST {{baseUrl}}/v3/users/1207/accounts
So now we know that the credit balance is 33 and from the /server/config
endpoint above, we know the server’s currency was USD.
In the Postman there are options to capture the requests being sent (top right) and display a console (bottom left) that shows the entire communication. There is also an option (top right) to display the code in various programming languages.
Recharge Credit
The token acquired earlier already contains the scopes needed (users credit
), but you can also request a new token with only these two scopes selected.
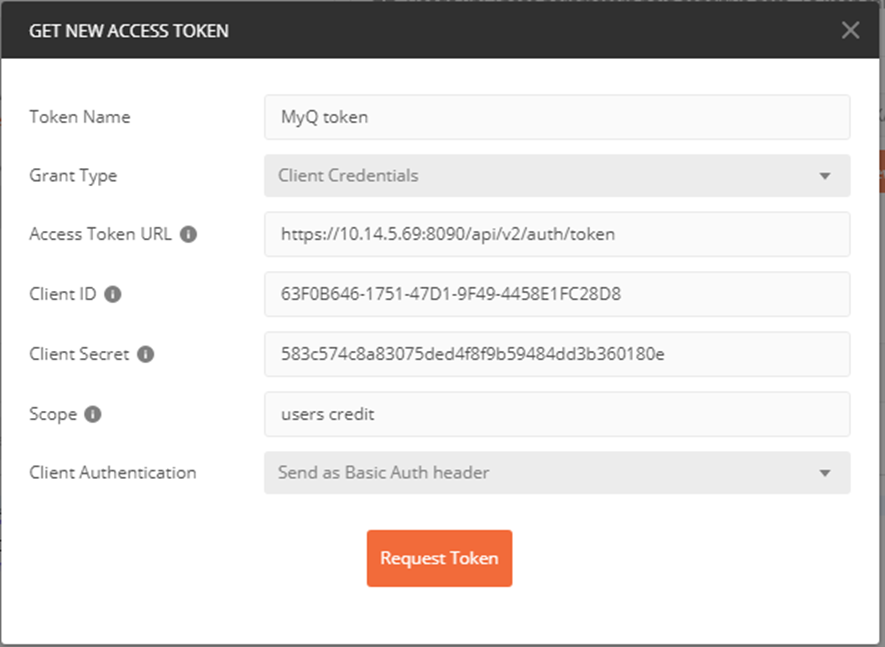
Firstly, obtain the user ID by calling the endpoint described earlier:
GET {{baseUrl}}/v3/users/find?username={{username}}
Required scope:
users
Example response:
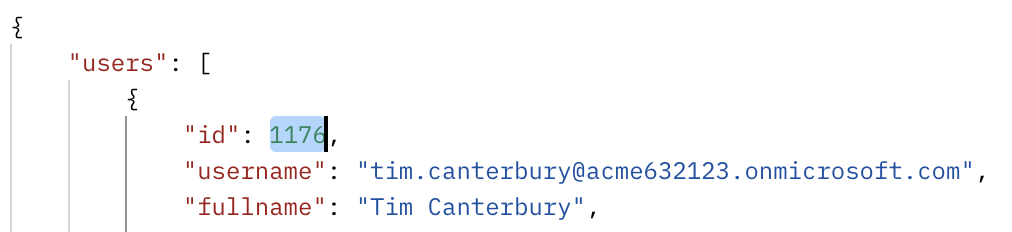
Then call a POST request to create a payment.
POST {{baseUrl}}/v3/rechargeProviders/{{paymentproviderreference}}/payments
Required scope:
credit
{{paymentproviderreference}}
useexternal
.To successfully call this endpoint, make sure the Content-Type is
application/json
.
In the body of the request, provide the additional information about – the user ID and the amount of money to recharge:
{
"userId": {{userid}},
"params": {
"amount": 13
}
}
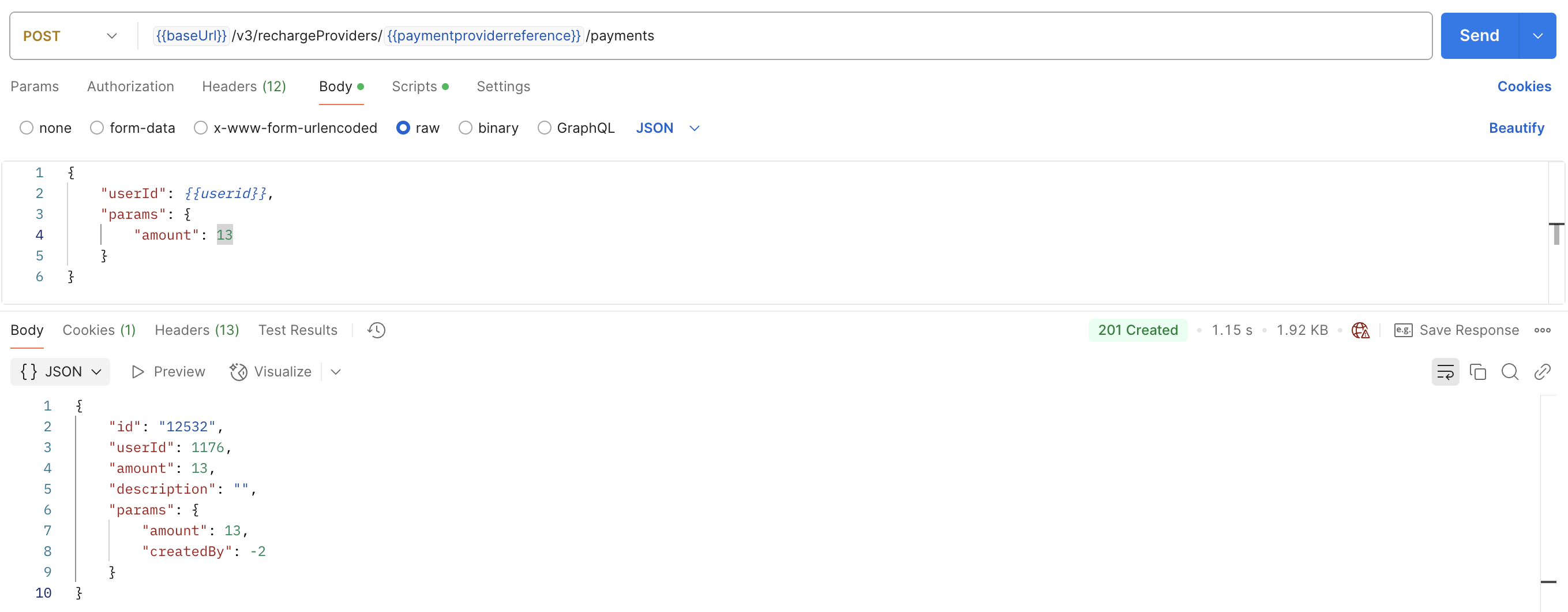
Example request:
POST {{baseUrl}}/v3/rechargeProviders/external/payments
{
"userId": 1176,
"params": {
"amount": 13
}
}
Make sure that External Payment Providers is enabled in MyQ X, in Settings > Credit.
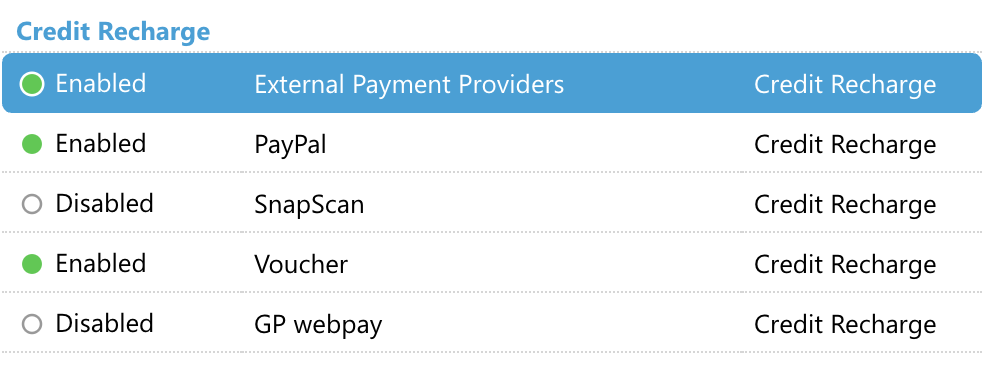
If it is not at the time of calling the endpoint to create a payment, you will receive a message: Invalid operation: The operation cannot be performed in the current state. Payment provider is disabled
.
The answer then returns the ID of the payment (12532
), we will use that to commit the payment.
Commit the payment by sending:
POST {{baseUrl}}/v3/rechargeProviders/{{paymentproviderreference}}/payments/{{paymentid}}/commit
Required scope:
credit
And finally, an answer is returned with the recharged amount.
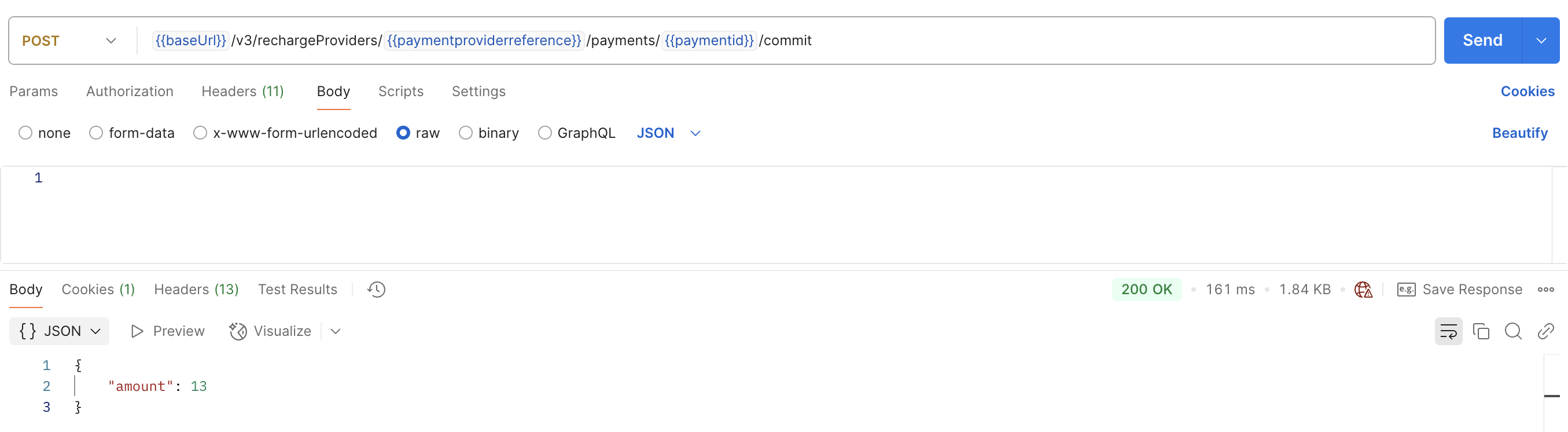
Example request:
POST {{baseUrl}}/v3/rechargeProviders/external/payments/12532/commit
And the user’s credit is topped up.

Since the API recharge does not support Snapscan or any other built-in payment provider except for vouchers, use the paymentProviderReference
called external
in your requests. So all payments will be visible in the logs with the source External Payment Provider.

User Authorization
In the steps above, we were using the client access token; this is a token that functions on behalf of your client application without the user context. However, users signing in to MyQ X and its applications need to obtain a user token to perform operations within their context.
Some endpoints require user token and cannot be called using the client token.
PIN, Password
To perform a sign in on behalf of the user, call the same endpoint as above:
https://serverHostname:webServerPort/api/auth/token
Replace the
serverHostname
andwebServerPort
with the values according to your installation.Use the Grant Type:
login_info
.Provide the information about a registered client in MyQ X that can interact with the REST API (
{{clientid}}
,{{secret}}
,{{userscopes}}
.In the
login_info
, specify the login type and PIN or password + username.
For more details, refer to our Online Help Center and read about supported login method types and their usage.
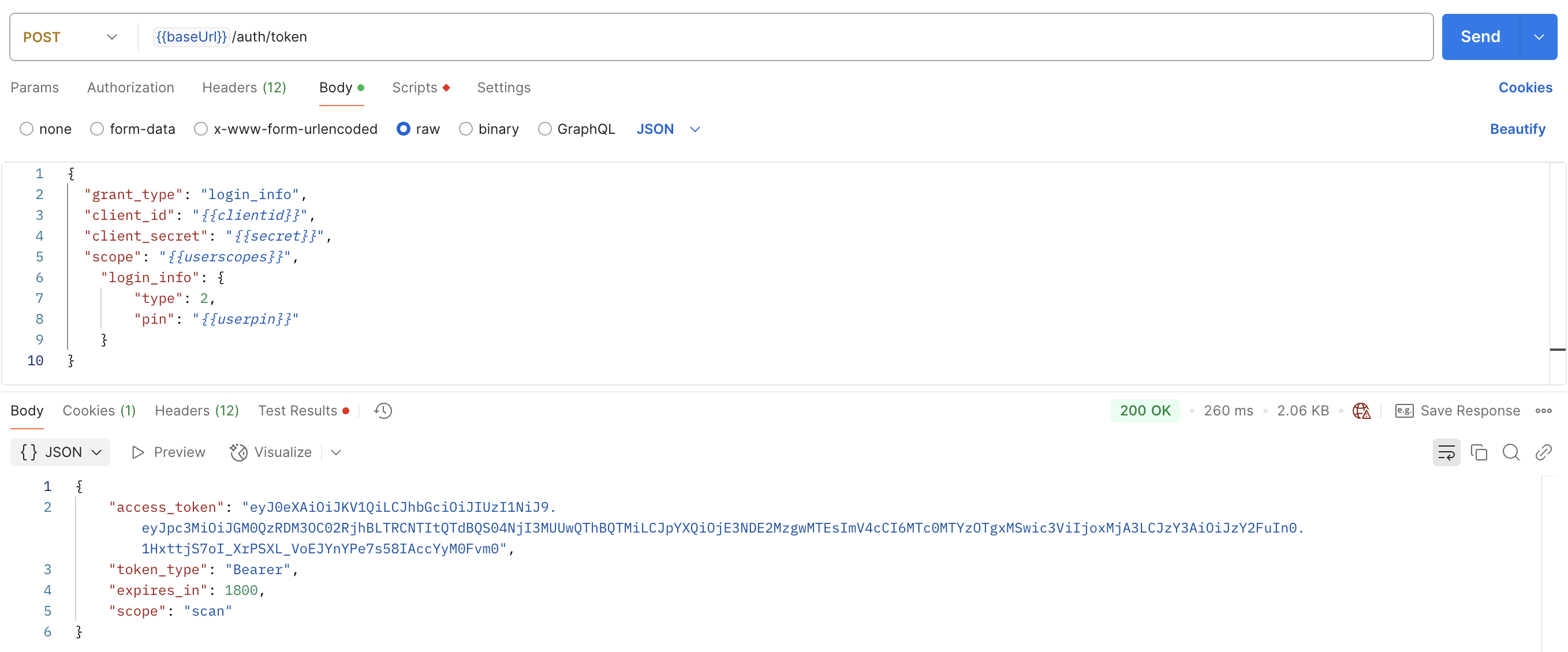
Example request:
POST https://print.acme.com:443/api/auth/token
{
"grant_type": "login_info",
"client_id": "F3D3D378-6F8A-4B52-A7AA-86271E0A8AA3",
"client_secret": "8c10ad3b3ed98ec08f56af721483679bcf6f7e81",
"scope": "scan",
"login_info": {
"type": 2,
"pin": "507875"
}
Example response:
{
"access_token":"eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJpc3MiOiJGM0QzRDM3OC02RjhBLTRCNTItQTdBQS04NjI3MUUwQThBQTMiLCJpYXQiOjE3NDE2MzgwMTEsImV4cCI6MTc0MTYzOTgxMSwic3ViIjoxMjA3LCJzY3AiOiJzY2FuIn0.1HxttjS7oI_XrPSXL_VoEJYnYPe7s58IAccYyM0Fvm0",
"token_type":"Bearer",
"expires_in":1800,
"scope":"scan"
}
Sign in with MyQ
MyQ X 10.1 and higher also support OAuth 2.0 authentication via Sign in with MyQ using the Grynt Type: authorization_code
.
You can use it to have users sign in with their MyQ X account, and obtain a user token to perform operations on their behalf.
For more details, refer to our Online Help Center and read about supported login method types and their usage.
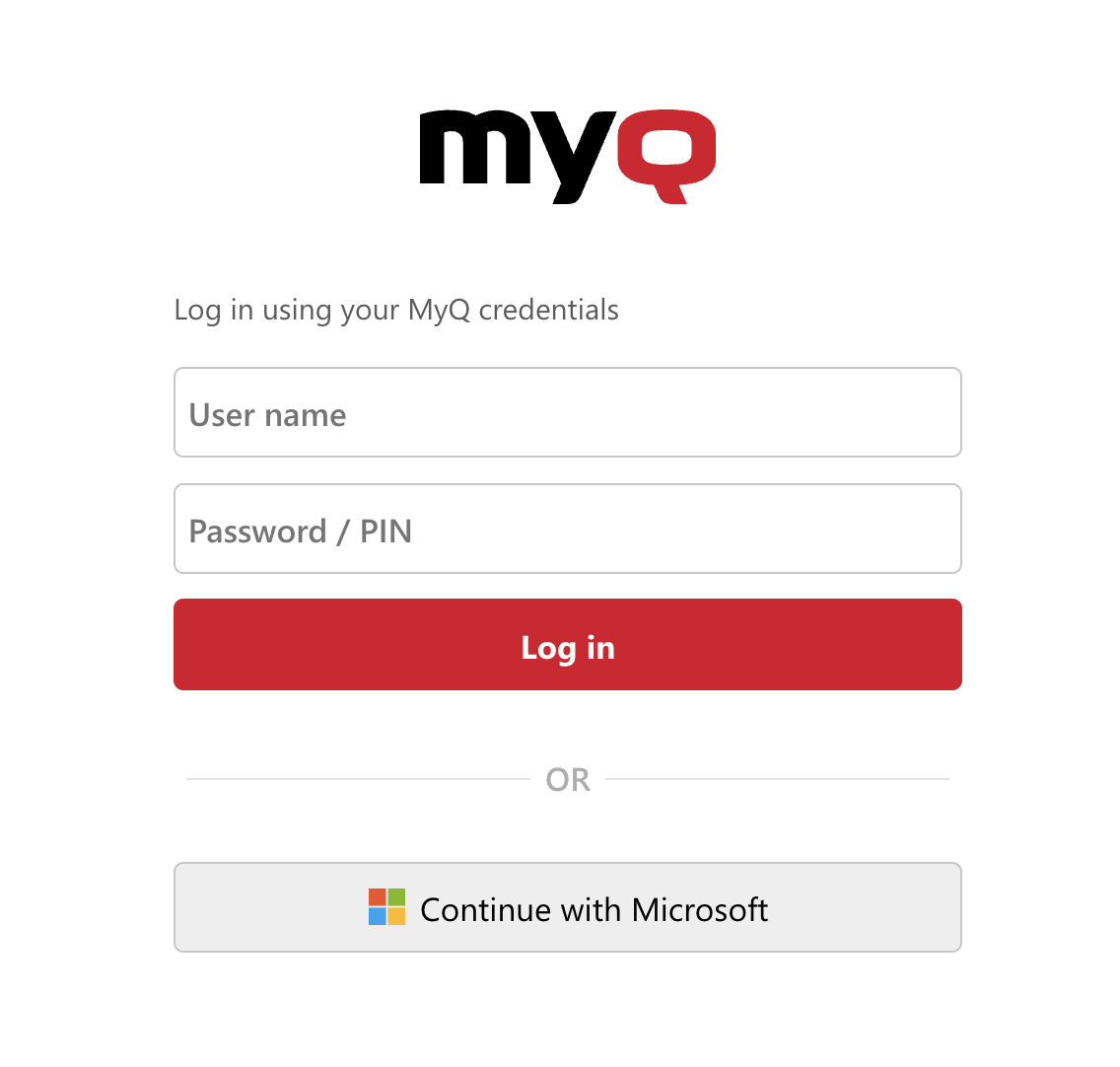
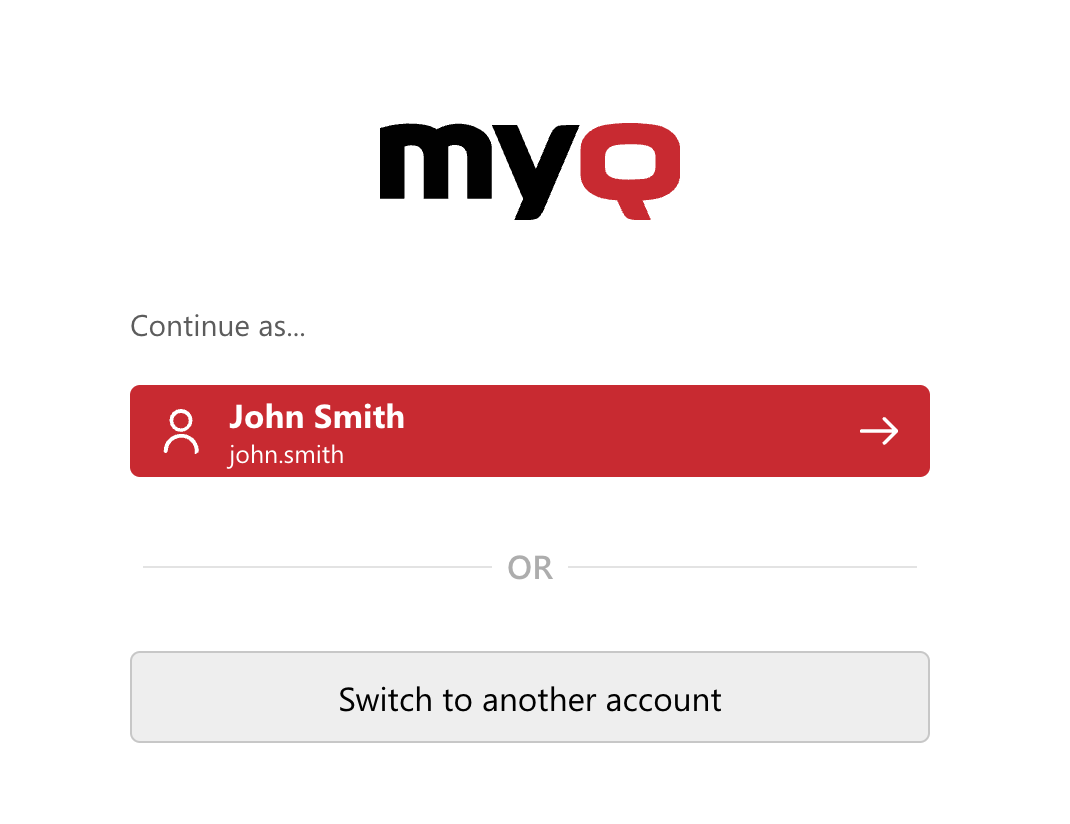
Additional Information
The REST API in MyQ X may change with time, and to ensure backward compatibility, its version can also be increased.
In versions MyQ X 8.2 and lower, the standard was
v1
.In MyQ X 8.2 and higher, versions
v2
andv3
with additional new endpoints are supported.The latest MyQ X 10.2 also supports both versions,
v2
andv3
.
Version v3
is recommended for general use since older versions may be deprecated in future MyQ X releases.
REST API versions between the Central Server and Print Server may differ, such as the available endpoints. Always follow the REST API docs for the product you intend to use.